Common Lisp the Language, 2nd Edition
Next: Numbers
Up: Common Lisp the Language
Previous: Overview of Syntax
Common Lisp provides a variety of types of data objects. It is important to
note that in Lisp it is data objects that are typed, not variables.
Any variable can have any Lisp object as its value.
(It is possible to make an explicit declaration that a variable will
in fact take on one of only a limited set of values. However, such
a declaration may always be omitted, and the program will still run correctly.
Such a declaration merely constitutes advice from the user
that may be useful in gaining efficiency. See declare.)
In Common Lisp, a data type is a (possibly infinite) set of
Lisp objects. Many Lisp objects belong to more than one
such set, and so it doesn't always make sense to ask what is the type
of an object; instead, one usually asks only whether an object belongs
to a given type. The predicate typep may be used to ask
whether an object belongs to a given type,
and the function type-of returns a type
to which a given object belongs.
The data types defined in Common Lisp are arranged into a hierarchy (actually
a partial order) defined by the subset relationship.
Certain sets of objects, such as the set of numbers or the
set of strings, are interesting enough to deserve labels.
Symbols are used for most
such labels (here, and throughout this book, the word ``symbol''
refers to atomic symbols, one kind of Lisp object,
elsewhere known as literal atoms). See chapter 4
for a complete description of type specifiers.
The set of all objects is specified
by the symbol t. The empty data type, which contains no objects, is
denoted by nil.

A type called common encompasses all the data
objects required by the Common Lisp language. A Common Lisp implementation
is free to provide other data types that are not subtypes of common.
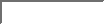
X3J13 voted in March 1989
(COMMON-TYPE)
to remove the type common (and the predicate commonp)
from the language, on the grounds that it has
not proved to be useful in practice and that it could be difficult to redefine in the
face of other changes to the Common Lisp type system (such as the introduction
of CLOS classes).
The following categories of Common Lisp objects are of particular interest:
numbers, characters, symbols, lists, arrays, structures, and functions.
There are others as well.
Some of these categories
have many subdivisions. There are also standard types defined to
be the union
of two or more of these categories. The categories listed above, while they
are data types, are neither more nor less ``real'' than other data types;
they simply constitute a particularly useful slice across
the type hierarchy for expository purposes.
Here are brief descriptions of various Common Lisp data types.
The remaining sections of this chapter go into more detail
and also describe notations for objects
of each type. Descriptions of Lisp functions that operate
on data objects of each type appear in later chapters.
-
Numbers are provided in various forms and representations.
Common Lisp provides a true integer data type: any integer,
positive or negative, has in principle a representation as a
Common Lisp data object, subject only to total memory limitations (rather than
machine word width).
A true rational data type is provided: the quotient of two integers,
if not an integer, is a ratio.
Floating-point numbers of various ranges and precisions are also
provided, as well as
Cartesian complex numbers.
-
Characters represent printed glyphs such as letters
or text formatting operations. Strings are one-dimensional
arrays of characters.
Common Lisp provides for a rich character set, including ways to
represent characters of various type styles.
-
Symbols (sometimes called atomic symbols for emphasis
or clarity) are named data objects. Lisp provides machinery
for locating a symbol object, given its name (in the form
of a string). Symbols have property lists, which in effect
allow symbols to be treated as record structures with an extensible
set of named components, each of which may be any Lisp object.
Symbols also serve to name functions and variables within programs.
-
Lists are sequences represented in the form of linked cells
called conses. There is a special object (the symbol nil)
that is the empty list. All other lists are built recursively by adding a new
element to the front of an existing list. This is done by
creating a new cons, which is an object having two components
called the car and the cdr. The car may hold anything,
and the cdr is made to point to the previously existing list.
(Conses may actually be used completely generally as two-element
record structures, but their most important use is to represent
lists.)
-
Arrays are dimensioned collections of objects.
An array can have any non-negative number of dimensions and is indexed
by a sequence of integers. A general array can have any Lisp object as
a component; other types of arrays are specialized for efficiency
and can hold only certain types of Lisp objects.
It is possible for two arrays, possibly with differing dimension information,
to share the same set of elements (such that modifying one array modifies
the other also) by causing one to be displaced to the other.
One-dimensional arrays of any kind are called vectors.
One-dimensional arrays of characters are called strings.
One-dimensional arrays of bits (that is, of integers whose values are 0 or 1)
are called bit-vectors.
-
Hash tables provide an efficient way of mapping any
Lisp object (a key) to an associated object.
-
Readtables are used to control the built-in expression parser
read.
-
Packages are collections of symbols that serve as name spaces.
The parser recognizes symbols by looking up character sequences
in the current package.
-
Pathnames represent names of files in a fairly implementation-independent
manner. They are used to interface to the external file system.
-
Streams represent sources or sinks of data, typically characters
or bytes. They are used to perform I/O, as well as for internal
purposes such as parsing strings.
-
Random-states are data structures used to encapsulate the state
of the built-in random-number generator.
-
Structures are user-defined record structures, objects that
have named components. The defstruct facility is used
to define new structure types. Some Common Lisp implementations may
choose to implement certain system-supplied data types,
such as bignums, readtables, streams,
hash tables, and pathnames, as structures,
but this fact will be invisible to the user.

- Functions are objects that can be invoked as procedures;
these may take arguments and return values. (All Lisp procedures
can be construed to return values and therefore every procedure is
a function.)
Such objects include compiled-functions (compiled code objects).
Some functions are represented as a list whose car is a particular
symbol such as lambda.
Symbols may also be used as functions.
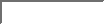
X3J13 voted in June 1988 (FUNCTION-TYPE)
to specify that symbols are not of type function,
but are automatically coerced to functions
in certain situations (see section 2.13).
X3J13 voted in June 1988
(CONDITION-SYSTEM)
to adopt the Common Lisp Condition System,
thereby introducing a new category of data objects:
-
Conditions are objects used to affect control flow in certain
conventional ways by means of signals and handlers that intercept those signals.
In particular, errors are signaled by raising particular conditions,
and errors may be trapped by establishing handlers for those conditions.
X3J13 voted in June 1988
(CLOS)
to adopt the Common Lisp Object System,
thereby introducing additional categories of data objects:
-
Classes determine the structure and behavior of other
objects, their instances. Every Common Lisp data object
belongs to some class. (In some ways the CLOS class system is
a generalization of the system of type specifiers of the first edition of this book,
but the class system augments the type system rather than supplanting it.)
-
Methods are chunks of code that operate on arguments
satisfying a particular pattern of classes. Methods are
not functions; they are not invoked directly on arguments
but instead are bundled into generic functions.
-
Generic functions are functions that contain, among other
information, a set of methods. When invoked, a generic function
executes a subset of its methods. The subset chosen for execution
depends in a specific way on the classes or identities of the arguments
to which it is applied.
These categories are not always mutually exclusive.
The required relationships among the various data types are
explained in more detail in section 2.15.
Next: Numbers
Up: Common Lisp the Language
Previous: Overview of Syntax
AI.Repository@cs.cmu.edu